Intro to Blocks
FORMAT & MIN ENGINE VERSION 1.21.90
This page discusses basic block features. You can learn more about other block components here.
NOTE
Vanilla blocks are hardcoded. You may not override or access them.
Minecraft Bedrock allows us to add custom blocks into our world with various vanilla-like properties. Custom blocks can have multiple stages (like plants), directional facing, and other useful features.
This tutorial will cover how to create basic blocks for the stable version of Minecraft.
Registering Blocks
Block definitions are structured similarly to entities: they contain a description and a list of components that defines the block's behavior.
Unlike entities, blocks do not have a resource definition other than in RP/blocks.json
.
Below is the minimum behavior-side code to get a custom block into the creative inventory.
{
"format_version": "1.21.90",
"minecraft:block": {
"description": {
"identifier": "wiki:custom_block",
"menu_category": {
"category": "construction", // The creative inventory or recipe book tab that the block is placed into
"group": "minecraft:itemGroup.name.concrete", // The expandable group that the block is a part of. (Optional)
"is_hidden_in_commands": false // Is the block hidden from use in commands? (Optional)
}
},
"components": {} // Must be here, even if empty!
}
}
Block Description
- Defines the block's
identifier
- a unique ID in the format ofnamespace:identifier
. - Configures which
menu_category
the block is placed into.- Also takes the optional parameters
group
andis_hidden_in_commands
.
- Also takes the optional parameters
The block description is also home to states and traits, which are covered in their own pages.
Adding Components
Right now, our custom block is using the default component values (which can be found here).
Let's configure our own functionality!
{
"format_version": "1.21.90",
"minecraft:block": {
"description": {
"identifier": "wiki:custom_block",
"menu_category": {
"category": "construction"
}
},
"components": {
"minecraft:destructible_by_mining": {
"seconds_to_destroy": 3
},
"minecraft:destructible_by_explosion": {
"explosion_resistance": 3
},
"minecraft:friction": 0.4,
"minecraft:map_color": "#ffffff",
"minecraft:light_dampening": 0,
"minecraft:light_emission": 4,
"minecraft:loot": "loot_tables/blocks/custom_block.json"
}
}
}
minecraft:destructible_by_mining
defines how long the player will need to mine the block until it breaks. Currently, it isn't possible to set different destroy times for different tools.minecraft:destructible_by_explosion
defines the resistance to explosions. The higher the value, the lower the chance of destruction.minecraft:friction
defines how much friction the block has. For example, soul sand has a high value for friction, so it slows the players. Ice has a lower friction value, so it has a slippery effect. The friction of classic blocks such as wood or stone is0.4
.minecraft:map_color
is the hex color code that will be displayed on a Minecraft map to represent this block.#ffffff
means white. You can get hex codes for other colors here.minecraft:light_dampening
defines how much light will be blocked from passing through.minecraft:light_emission
defines the light level the block will output.minecraft:loot
defines a loot table path for the block to drop. If this is removed, then the block will drop itself. You can learn more about loot tables here.
Browse more block components here!
Applying Textures
The geometry and material instances components should be used to determine how your block looks.
For our basic 16×16×16 pixel block, the vanilla model minecraft:geometry.full_block
will be used.
"minecraft:geometry": "minecraft:geometry.full_block",
"minecraft:material_instances": {
"*": {
"texture": "wiki:custom_block"
}
}
Now, we need to link the texture shortname to an image file path in RP/textures/terrain_texture.json
:
{
"resource_pack_name": "wiki",
"texture_name": "atlas.terrain",
"texture_data": {
// Our texture shortname:
"wiki:custom_block": {
"textures": "textures/blocks/custom_block" // Link to an image file name
}
}
}
Per-Face Textures
Textures can also be applied per face. For example, a custom "compass block" could use the following ✨stunning✨ textures:
textures/blocks/compass_block_down.png
textures/blocks/compass_block_up.png
textures/blocks/compass_block_north.png
textures/blocks/compass_block_east.png
textures/blocks/compass_block_south.png
textures/blocks/compass_block_west.png
The material instances should look like this:
"minecraft:material_instances": {
"down": {
"texture": "wiki:compass_block_down" // This texture appears in destruction particles
},
"up": {
"texture": "wiki:compass_block_up"
},
"north": {
"texture": "wiki:compass_block_north"
},
"east": {
"texture": "wiki:compass_block_east"
},
"south": {
"texture": "wiki:compass_block_south"
},
"west": {
"texture": "wiki:compass_block_west"
}
}
With the following terrain_texture.json
data:
{
"resource_pack_name": "wiki",
"texture_name": "atlas.terrain",
"texture_data": {
"wiki:compass_block_down": {
"textures": "textures/blocks/compass_block_down"
},
"wiki:compass_block_up": {
"textures": "textures/blocks/compass_block_up"
},
"wiki:compass_block_north": {
"textures": "textures/blocks/compass_block_north"
},
"wiki:compass_block_east": {
"textures": "textures/blocks/compass_block_east"
},
"wiki:compass_block_west": {
"textures": "textures/blocks/compass_block_west"
},
"wiki:compass_block_south": {
"textures": "textures/blocks/compass_block_south"
}
}
}
Applying Sounds
The mining sound, step on sound, breaking sound, and placement sound of custom blocks can be determined by the sound
parameter in RP/blocks.json
.
Learn more about block sounds here!
{
"format_version": "1.21.40",
"wiki:custom_block": {
"sound": "grass"
}
}
Defining Names
Finally, let's define our block names like this:
tile.wiki:custom_block.name=Custom Block
tile.wiki:compass_block.name=Compass Block
You can learn more about translation here.
Result
In this page, you've learnt about the following:
...but it's only the beginning, see what else you could do below!
What's Next?
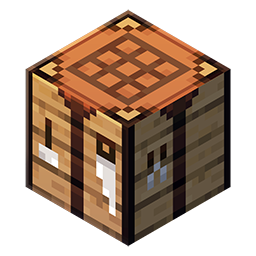
Add Functionality
Learn about the available block components to craft unique gameplay.
Why not give your block a custom model with the geometry component? You could also configure your own collision and selection boxes to match!
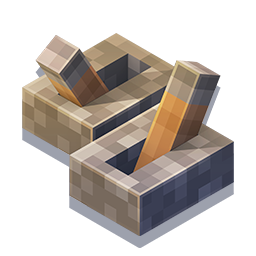
Create Variants
Make use of block states and permutations to conditionally enable components on your blocks.
For example, you could add liquid depth levels to your custom tank block, with support for multiple liquid types.
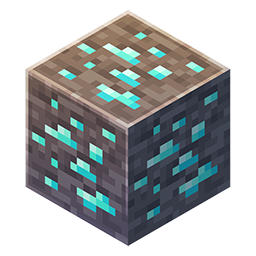
Replicate Vanilla
Browse several complete replicas of existing blocks in the Vanilla Re-Creations category.
Start simple with custom glass blocks, making use of material instances!